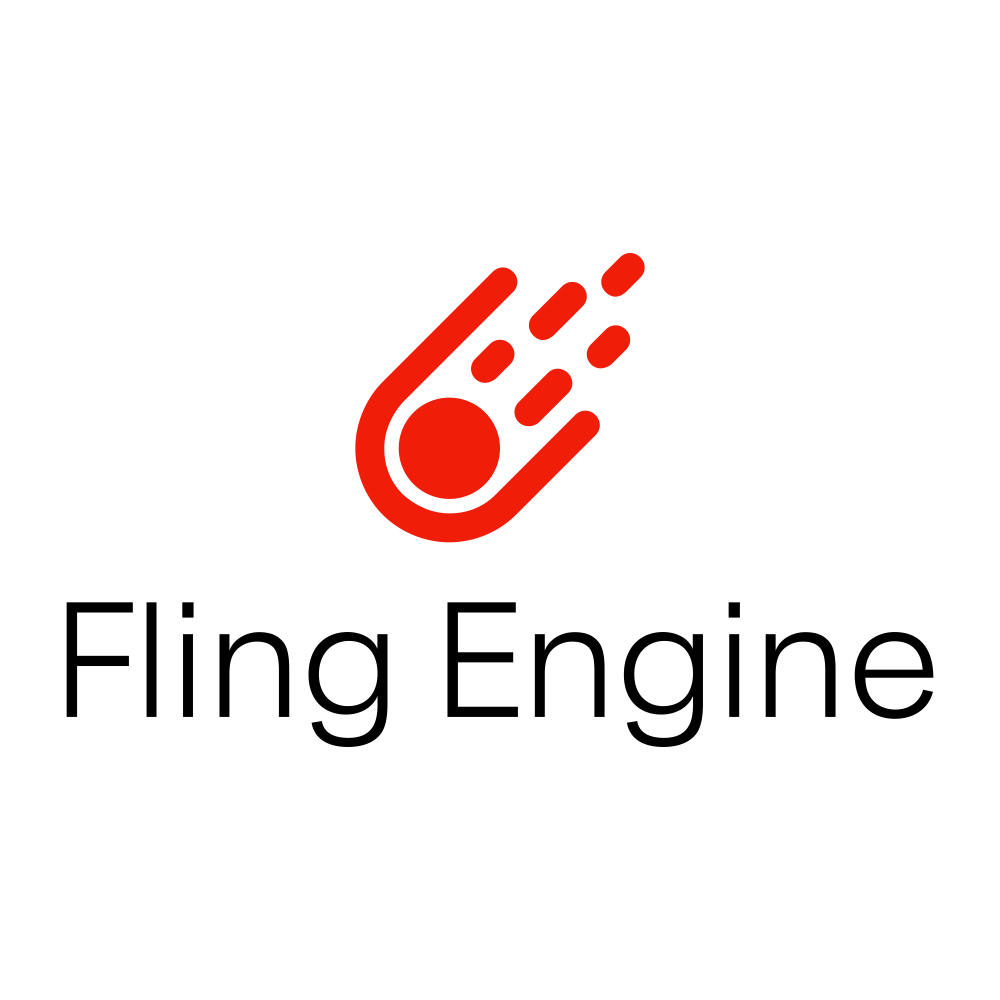
Scalable
With the power of Vulkan and a data oriented architecture the engine can get the most out of your hardware. Our memory model is flat, which makes Parallelization of our pipeline easy while keeping as much cache coherency as possible.
Extendable
The engine is completely open source and all code is peer reviewed and tested before being submitted. This helps us maintain a high quality bar while ensuring that the code is scalable and easy to understand.
Simple
The framework for the engine is written in such a way that all you need to do to create a game in Fling is create a game class. From there you have all the control to write whatever kind of gameplay systems you want.
Usage
All you need to do in order to make a game with the Fling Engine is
to override the Fling::Game
class, and specify your game when you
create your engine instance.
Here is a very simple example of a game's main.cpp
:
#include "FlingEngine.h"
class YourGame : public Fling::Game
{
/**
* Called before the first gameplay loop tick.
* Do any initialization for custom gameplay systems here.
*/
void Init(entt::registry& t_Reg) override {}
/* Called when the engine is shutting down */
void Shutdown(entt::registry& t_Reg) override {}
/**
* Update is called every frame. Call any system updates for
* your gameplay systems inside of here
*/
void Update(entt::registry& t_Reg, float DeltaTime) override {}
};
/* Entry point for using the Fling Engine! */
int main(int argc, char* argv[])
{
Fling::Engine Engine = {};
try
{
Engine.Run<YourGame>();
}
catch (const std::exception& e)
{
std::cerr << e.what() << std::endl;
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
Building from Source
The Fling Engine uses CMake for quick and easy setup of your development environment no matter what platform you are on.
There are several CMake options to consider when building the Fling Engine:
-DCMAKE_BUILD_TYPE=Release
will compile your code in release mode (optimizations on), but keep logging enabled and the asset directories absolute.-DDEFINE_SHIPPING=ON
will compile your code in release mode, but the Assets and Config directories are now relative to the executable.
The Assets Folder
The Assets folder is where all assets (models, textures, shaders, levels, etc) should be placed. When you run CMake file paths are generated to be absolute based on where you have cloned the repo. This means that if you move the folder or repo, you must re-run CMake.
A simple setup like this makes quick iteration on assets much easier without the overhead of needing a full asset management database.
Obviously you will want asset paths to be dynamic for a shipping product. All you have to do
in order to accomplish this is to set the -DDEFINE_SHIPPING
CMake flag to be ON
when you run CMake. This also adds a C++ define
that you can check simply:
#ifdef FLING_SHIPPING
// Do some nice stuff
#else
// Do non-shipping code, perhaps with a lot of log messages
#endif
The Config Folder
Fling Engine uses some simple ini
files for settings that we thought might be
useful for you to tweak. Check out a full config
here.
Here is a quick example config file:
; Core engine config example
[Engine]
WindowWidth=800
WindowHeight=600
; The text shown in the window title bar!
WindowTitle=Fling Engine
; Will show what git branch and commit head in the title bar
DisplayBuildInfoInTitle=true
DisplayVersionInfoInTitle=true
; This is relative to the Assets directory
WindowIconImage=FlingEngineLogo.png
; Graphics API Settings
[Vulkan]
EnableValidationLayers=false
;UI settings
[Imgui]
display=true
[Camera]
MoveSpeed=10
RotationSpeed=40
; Game settings! Add any custom config file settings you want to read in here
; @see FlingConfig
[Game]
; Start level is relative to the assets directory
; @see World::LoadLevel
StartLevel=Levels/EmptyLevel.json